Type-Checking React and Redux (+Thunk) With Flow — Part 2
In short
In this article, we’ll cover how to type-check Redux. We have very simple counter app which we’re going to type-check. We are also using the thunk middleware for Redux. Things may vary when you have a more complex project or use different middleware. Keep in mind that this is the result of learning and experimenting. You might need to tweak it for your workflow and taste.
Install type definitions
The first thing we need to do is to install the type definitions for Redux and and React Redux. Thanks to flow-typed, they are just one command away.
Install flow-typed if you haven’t done that yet, then cd to your project directory and type:
This will automatically find and install type definitions for Redux, React Redux and other libraries specified in your package.json.
Make sure you’ve installed flow-bin locally from NPM first.
Type-check actions
We’ll declare types for all actions in a your project and export a single Action type. Declaring types for all the actions might seem a little bit of work, and you might be tempted to define a generic type like { type: string, payload?: any }, but declaring specific types will help to find mistakes both in your action type strings and shape of payload.
Note that it also depends on what other libraries you use. If you use libraries which generate actions for you, then this list can get out of date quickly. If the library always generates a specific shape of action, you can probably define a generic type for it.
Type-check reducers
We can define the reducer normally with type annotations and it’ll mostly work:
This will have basic type safety, but still fragile, because flow can infer the type of the action from action.type, but if you make a typo in action.type, then it’ll infer the type as any, basically bypassing type check.
To avoid this scenario, we can use a helper similar to what’s described in Redux docs:
Our helper can look like this:
Note that this doesn’t prevent you from typos in action names. But unlike switch statements, trying to access non-existing properties on the action object gives a warning since actions will no longer be inferred. In addition, flow can no longer refine type of the action according to the action type, so it has a different set of tradeoffs.
You could also define a ActionType enum with all the actions and replace [key: string] in above helper with [key: ActionType] to catch typos.
Though it’s repetitive given that we already have an enum with all possible actions.
Type-check state
To make it easier to define the type for the redux state, we need to do some changes in the root reducer. Basically, move the reducer map to a separate object and export the Reducers type.
Thanks to this great tip from Adam Miskiewicz, we can automatically generate the types for our state from our reducers.
Isn’t it magic? Yes, yes, it is.
What I love about this approach is, your reducers describe your state, both for Redux and for Flow. You don’t have to type out the shape of your state separately for flow, which is great because it will never get out of date.
Type-check store
To define types for the store, we can import the Store and Dispatch from the installed definitions, and then set them up with our State and Actiontypes.
Since we’re using the redux-thunk middleware, our Dispatch method will be little different than the default method, i.e. — we need to support dispatching thunks along with plain actions.
Now we just need to annotate the store with our shiny new type:
Type-check action creators
Action creators are just simple functions, so we just need to annotate them with the types we created before:
Flow will warn when you’re returning invalid actions from the action creator, or trying to dispatch an invalid action.

We don’t necessarily have to use action creators. Since we’ve typed our store above, we could easily dispatch plain actions.
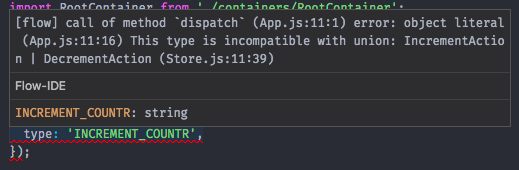
Type-check containers
The last bit of the puzzle is type-checking the container components. We just need to annotate mapStateToProps with the State type we created earlier.
Combine with the react-redux type definitions we installed earlier, it gives proper type-checking for the props we’re passing to the underlying component.

For example, if we forget to pass a prop, or pass a prop of invalid type in mapStateToProps or mapDispatchToProps other than what’s specified in the Counter component, Flow will warn us.
Wrapping up
That’s it. We now have a decent type-checked React and Redux codebase; you can check the complete React and Redux project.
There’s so much more to learn and experiment, but I hope this gave you a good starting point. Do you have any tips on how to improve this setup?