In my daily job, I see various codebases and collaborate with a lot of developers from all over the world. I’ve noticed that many programmers don’t pay enough attention to things like code cleanness, tests or typings. All of those things are “optional” in JavaScript so you can develop mobile apps without them, but it’s not the way to go if you’re collaborating with other devs or if your project grows larger. There are a few tools built around React which can help you improve your code quality in no time. I’ll try to briefly describe them here and give you a small boilerplate with all of them combined and set up. You can find the link at the end of this article.
ESlint
ESlint is a very basic tool which provides linting utility for modern JavaScript. It’s very pluggable so you can install it globally on your machine and set it up in your IDE to have real-time checks or just simply attach it to your project. For sure the last approach is better because you can have a version targeted especially for your project and also run this kind of check on continuous integration systems. It’s very simple to start with ESlint. First you need to add it to your project:
NOTE: You can use npm instead.
ESlint requires configuration placed inside .eslintrc file, or under “eslintConfig” property in your package.json. If you don’t want to spend time defining all the rules for your project, I recommend the eslint-config-airbnb package, which contains very good rules for writing good React applications. Here’s how it’s done:
Now you should see peerDependencies versions listed within a JS object inside your console. Simply replace the hashes in the version and run:
Within the configuration file, create an “extend” key and add airbnb to an array of extensions, and you’re ready to go!
<p-bg-col>NOTE: You can override some of the rules from this preset inside your config.<p-bg-col>
Prettier
While ESlint is a great tool to analyze your code and eliminate various bugs and bad code, there is another solution with the power to unify your code style on each file with a single command. Prettier, a great tool released by Christopher Chedeau (@vjeux) and James Long (@jlongster), is an opinionated JavaScript formatter with advanced support for language features from ES2017, JSX, and Flow. It removes all original styling and ensures that all outputted JavaScript conforms to a consistent style. Like ESlint, it has plugins for various IDEs, so you can enable it when saving each file. If you don’t want to do this you can just run a CLI command or hook it to pre-commit. It’s very easy to start:
yarn add prettier --dev
When prettier is available in your project you can add a new script to your npm scripts in package.json; the entry could look like this:
It tells prettier to format your .js files within the src/folder with some formatting options. You can check the full GitHub docs.
Prettier plays along with ESlint very well — it provides some eslint presets, so if you’re using them both, don’t forget to add extensions, just like you did with the airbnb preset:
and then:
Flowtype
I’ve begun my programming journey with Java. When I moved to web development, I loved the freedom JavaScript gives in everyday development, but I felt like something was missing. Everything was very well typed in Java apps, and when I heard about Flow I was very excited. Static typing helps you eliminate a lot of errors and makes the code really readable for other developers. What’s great about Flow is the fact that it plays along with your IDE in real time, just like ESlint. In React Native the .flowconfig file isalready present in the generated app. You simply have to install flow:
To perform typecheck in your app just add the script to your package.json
Then you can run:
If you want to add Flow to your web application you have to specify .flowconfig by yourself. But don’t worry — it’s super easy, just follow the Flow docs and read the article about the configuration.
Haul
If you haven’t heard about it yet, Haul is an open-source library which is a replacement for react-native packager. It allows you to access a full webpack ecosystem, so things like aliases or symlinks are easily doable. To get more info about what’s possible with Haul, please check the haul repo and the webpack docs. I’ll quickly show you how to add Haul into your project and set some aliases to avoid relative paths and make your code a bit more readable for other developers. First, you need to add haul into your project:
NOTE: It used to be haul-cli until June 2017.
From now on you can run the haul init script:
Notice that there is a new file called webpack.haul.js generated within your project. It provides a hook which allows you to mutate Haul’s default webpack config. There are tons of options to customize your project with webpack. I’ll show you real quick one of my favorite options, which are aliases for directories in your project. Open your webpack.haul.js and edit the exported function:
In this specific case, I created aliases for components, containers, and stores directories and to the routes file.
Now within your project, you can import components like this:
Amazing, isn’t it?
If you’re using Flow inside your project, aliases will produce an error. If you want to get rid of it, you need to specify module.name_mapper in your .flowconfig options. The module name mapper definition for our aliases should look like this:
No errors! Yay!
UPDATE: Recently, we've refreshed and add new features to Haul. As it was a major update, we decided to rename the library to Re.Pack. Check out its webpage to see what's new!
Also, we've prepared an episode of The React Native Show podcast fully dedicated to Re.Pack in which Paweł Trysła, the lead engineer at Re.Pack (previously Haul) dives into details of this library. You can watch it here:
Jest
Testing is very important to ensure your code quality. When it comes to testing React components, Jest is my first call. It’s already bundled with the RN initial project, so you don’t have to install anything. With snapshot testing, you can cover a nice amount of your code without writing a lot of code in your test files. Look how simple wring a test for the component is:
If you want to learn more about snapshot testing check out this great Callstack article.
Other tools
There are a lot of other tools and libraries to help you increase your app development skills. I put everything you read today and some other cool libs inside an example app. If you want to learn more about other tools, check how to write React Native apps with MobX.
Mike.
Learn more about
Developer Experience
Here's everything we published recently on this topic.
We can help you move
it forward!
At Callstack, we work with companies big and small, pushing React Native everyday.
React Native Performance Optimization
Improve React Native apps speed and efficiency through targeted performance enhancements.
Super App Development
Turn your application into a secure platform with independent cross-platform mini apps, developed both internally and externally.
Mobile App Development
Launch on both Android and iOS with single codebase, keeping high-performance and platform-specific UX.
React Development
Develop high-performance React applications with advanced patterns and scalable architectures.
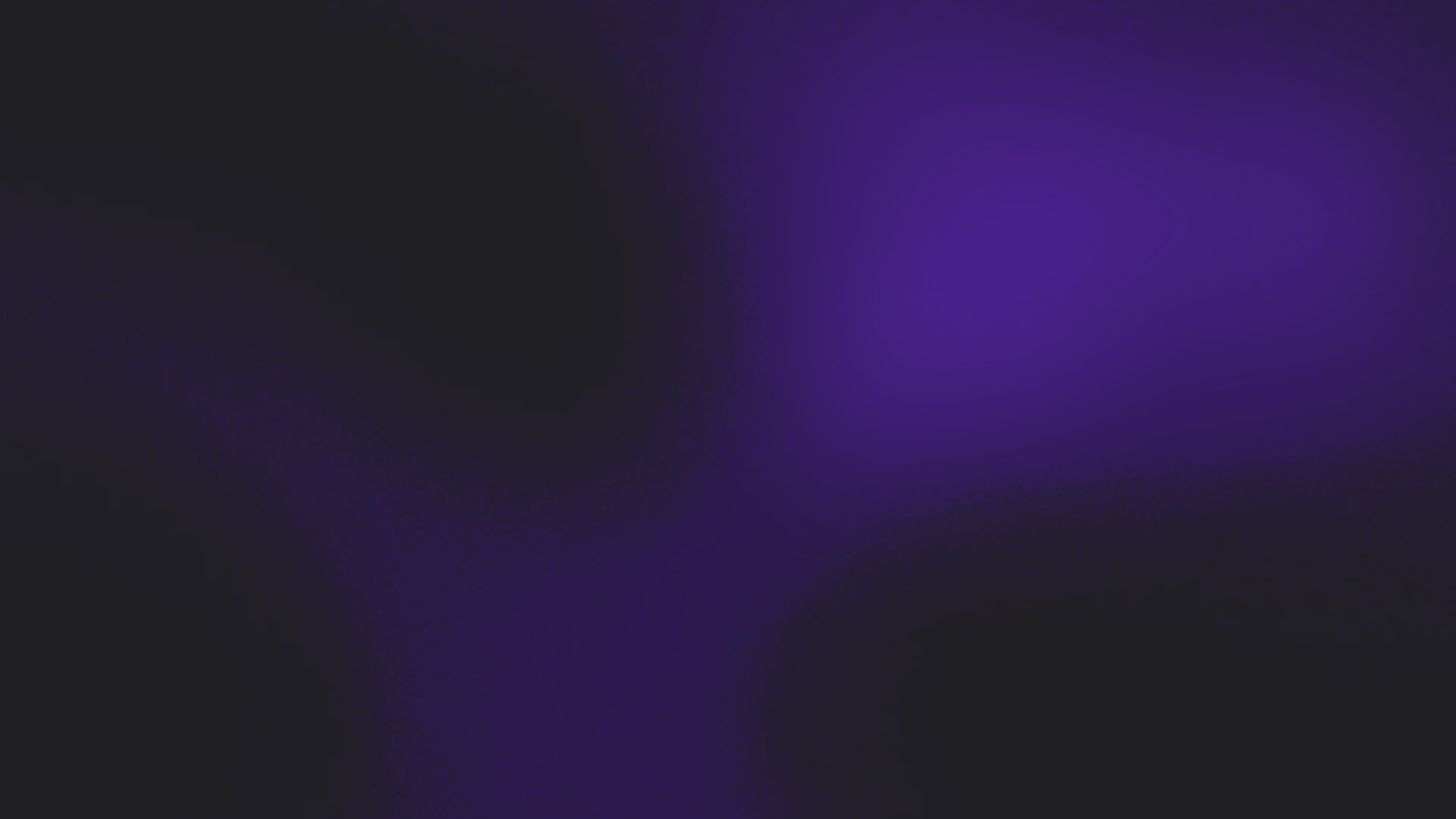