Writing a Native Module for React Native Using Kotlin
Trying out new techniques and technologies is great, so why don’t we try out Kotlin for React Native modules?
We’re about to build a react-native-utube-player, a Native UI component that allows playing YouTube videos in our React Native app. We’re going to achieve this by using an existing Android library, android-youtube-player, created by Pierfrancesco Soffritti.
Kotlin dependencies
Kotlin is fully interoperable with Java, meaning they can call one another and coexist in one project. This gives us a great opportunity to try it out in React Native. To get started, we have to add Kotlin dependencies to our library’s build.gradle. After that, we’re ready to write some Kotlin!
Project structure
Starting from the ground up, we create a basic structure for our library.

Note: We keep standard android structure, with Java directory in mainsource dir, so that React Native Linker can easily find our module and link it to project.
Minimal content for a valid manifest file:
Gradle setup
To build an Android module, we have to use the android-libraryplugin, alongside with kotlin-android. Let’s add those to buildscript blockand apply them.
The last step is to add Android settings block and the rest of the module dependencies. Here’s an example of finished build.gradle file:
Now we’re ready to do some coding. To make a valid Native Module, we have to create two classes, one extending ReactPackage, providing information about the capabilities of the library, and another one, SimpleViewManager, to draw our widget. Let’s get into it.
ReactPackage implementation
This class provides a list of functionalities and UI components for usage in React Native. We have to override two methods:
createNativeModules — returns a list of instances of NativeModule class, which defines functions that can be called from JavaScript. We’ll not be using this one today.
createViewManagers — this function returns a list of Native UI components, instances of ViewManager. Implementation of this class is a birthplace of our widget.
UTubePackage.kt implementation
ViewManager implementation
We’ll implement SimpleViewManager, which is a convenient subclass of ViewManager — it applies common properties such as background color, opacity, and Flexbox layout.
In our class, we’re required to override two methods from the interface:
getName: a unique name for our component — will be used to import it on JavaScript side
createViewInstance: this one requires to return an instance of our view implementation.
To get YouTubePlayerView instance, we pass reactContext as the only argument to it a constructor. Call initialize method on it and pass a lambda function, which acts as a callback, called when View is ready to use. It receives YoutubePlayer instance as an implicit argument it.We use this one to load videos into our player.
UTubeView.kt implementation
To get data in React way, we need to be able to read props passed to a component. Let’s create a function for will be fired whenever there’d be a change in UI tree.
setVideoId method is annotated with @ReactProp, meaning it will be fired with new prop value (named videoId) of type String? (String or null). If the prop is not the same and exists, we use it to load our video.
That’s it! We can now use our widget in React Native, so let’s write a consumer for it.
To receive a reference to our widget, we’ll call requireNativeComponent with its name (the one you specify in overridden getName method). We require three props to make it work — width, height, and videoId. Otherwise, our component won’t show or play at all.
Great, all set! We can import it now into our app and play some videos!
Use module in our app
Now, we’re ready to use a module in our app. Let’s import it into our view tree and see it in action.
And this is the outcome:
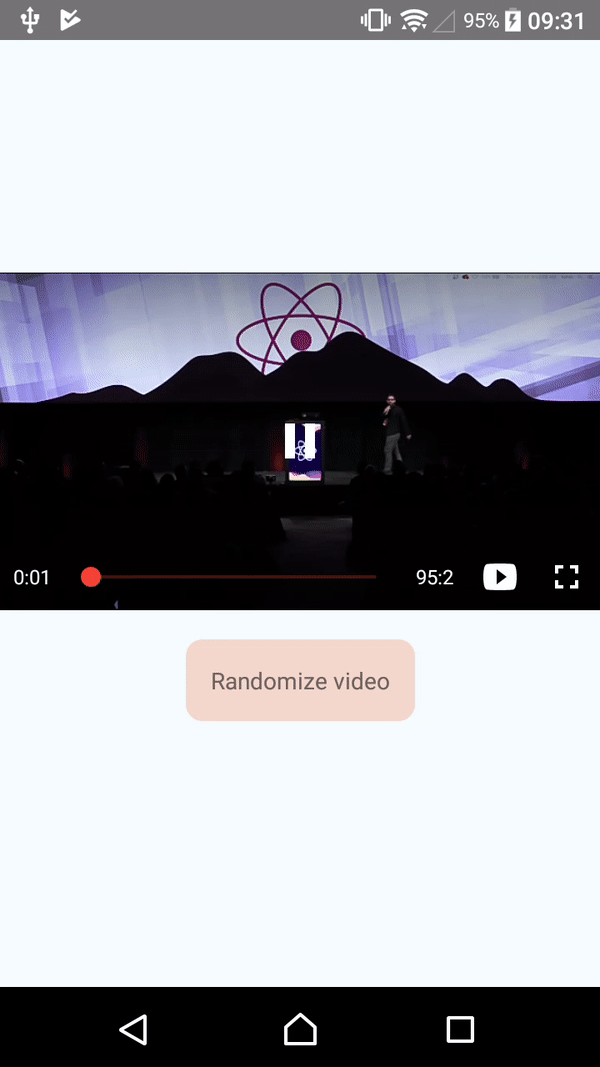
Final words
I hope you enjoy this quick entry point to Kotlin in React Native. There is still big room for improvement, but we came to a nice outcome with no big effort. Full code is hosted at GitHub, so you can try it out on your own.
Learn more about
Android
Here's everything we published recently on this topic.
We can help you move
it forward!
At Callstack, we work with companies big and small, pushing React Native everyday.
React Native Performance Optimization
Improve React Native apps speed and efficiency through targeted performance enhancements.
Release Process Optimization
Ship faster with optimized CI/CD pipelines, automated deployments, and scalable release workflows for React Native apps.
React Native Brownfield
Integrate React Native into existing native application and start sharing code across platforms immediately.
Mobile App Development
Launch on both Android and iOS with single codebase, keeping high-performance and platform-specific UX.
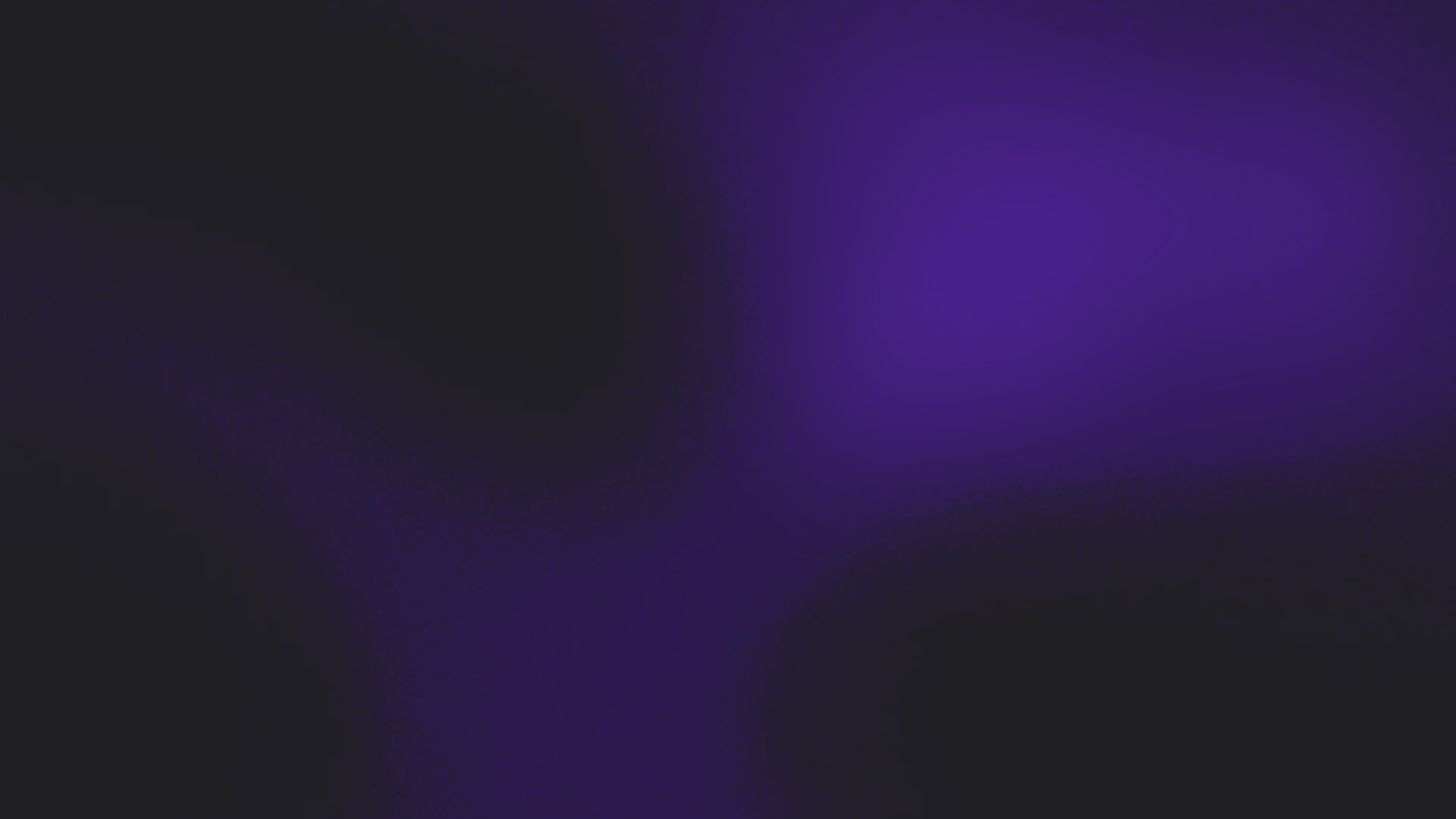