Reusable React Native Components
For most people who are new to React Native it’s pretty hard to find simple methods to customize components that could be reused within the app. This article will help you create more efficient code with less effort during development process.
Some basics at the beginning. There are three main patterns to customize components
- Pass data via props
- Nest component
- Higher order component
Let’s go through them using a simple button component as an example. It’s really important to choose best solution depending on the use case and make them more reusable because it will save your time and allow you write less code.
1. Pass data via props
First method is the most common. While creating component we need to think about best setup of props that can be reused with different values. I’ll explain it on <Button /> example. Our basic component has initial values set in props, label with text displayed on the button, buttonStyle with style of component, textColor for style of text, onPress for an action on button press.
<Button /> is ready to use in multiple places, but if we want adapt it for a different use case we can simply change its behavior via props. It happens pretty often that we have to modify styles or text a bit in the other view.
First button is our basic one which will be used the most, but for some other scenario we might want to display it with different look, so changing it via props is the best solution.
2. Nest component
This method is useful if we want to reuse a variant of the button in several places. The problem is we have to override the styles in every place we want to use them. It simply comes to copy-pasting the code. We can write the variant as a new component with overrides already applied, and then reuse that. Lets create a function in Button.js with onPress argument passed from container and other props defined inside.
For now if we want to use brown button in several places, much simpler!
Just import the component where you want to use it.
import Button from '/Button';
and call for it in return
3. Higher order component
Higher order components can be used to enhance another component with a new behaviour. HOCs are basically the functions which return a new component wrapping another component, i.e. I’ll add simple animation on one of the buttons instead of multiplying animations in buttons. Let’s add a simple scale animation on the button when pressed.
Since we’re passing a custom onPress, we need to take care of calling the onPress prop passed by the user in callback of animation function. In render method we must separate onPress from rest of props. So the old one will be overwritten by new onPress. Action of button will start right after animation and other props are passed to wrapped component. To keep our code clean, I prefer to use HOC’s inside of components code. In Button.js we just pass to function any of buttons as the argument.
In container we can use it like any other component
On emulator it looks like this:
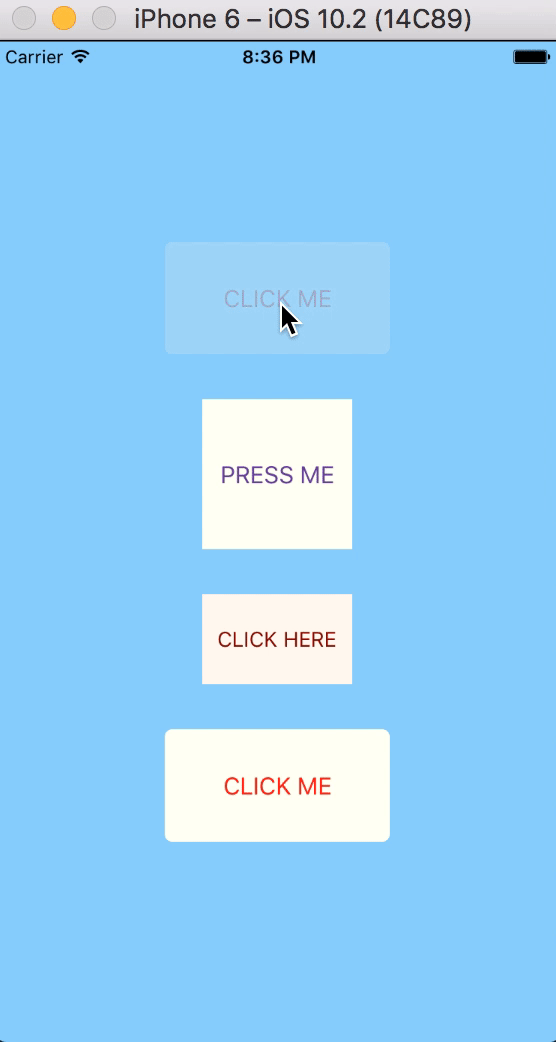
I hope that after reading this post you learned about optimization of code and making your component reusable. It’s important to think wider about small elements, if they are unique or more common, their variations within the app and so on… With good choices life can be much easier and tasks less time-consuming.
There's a github repo with example.
Learn more about
Scalability
Here's everything we published recently on this topic.
We can help you move
it forward!
At Callstack, we work with companies big and small, pushing React Native everyday.
Quality Assurance
Combine automated and manual testing with CI/CD integration to catch issues early and deliver reliable React Native releases.
Scalability Engineering
Design and validate React Native architectures that scale-supporting high traffic, modular teams, and long-term performance.
Super App Development
Turn your application into a secure platform with independent cross-platform mini apps, developed both internally and externally.
Module Federation Development
Use Module Federation to provide dynamic features within React Native mobile applications post-release.
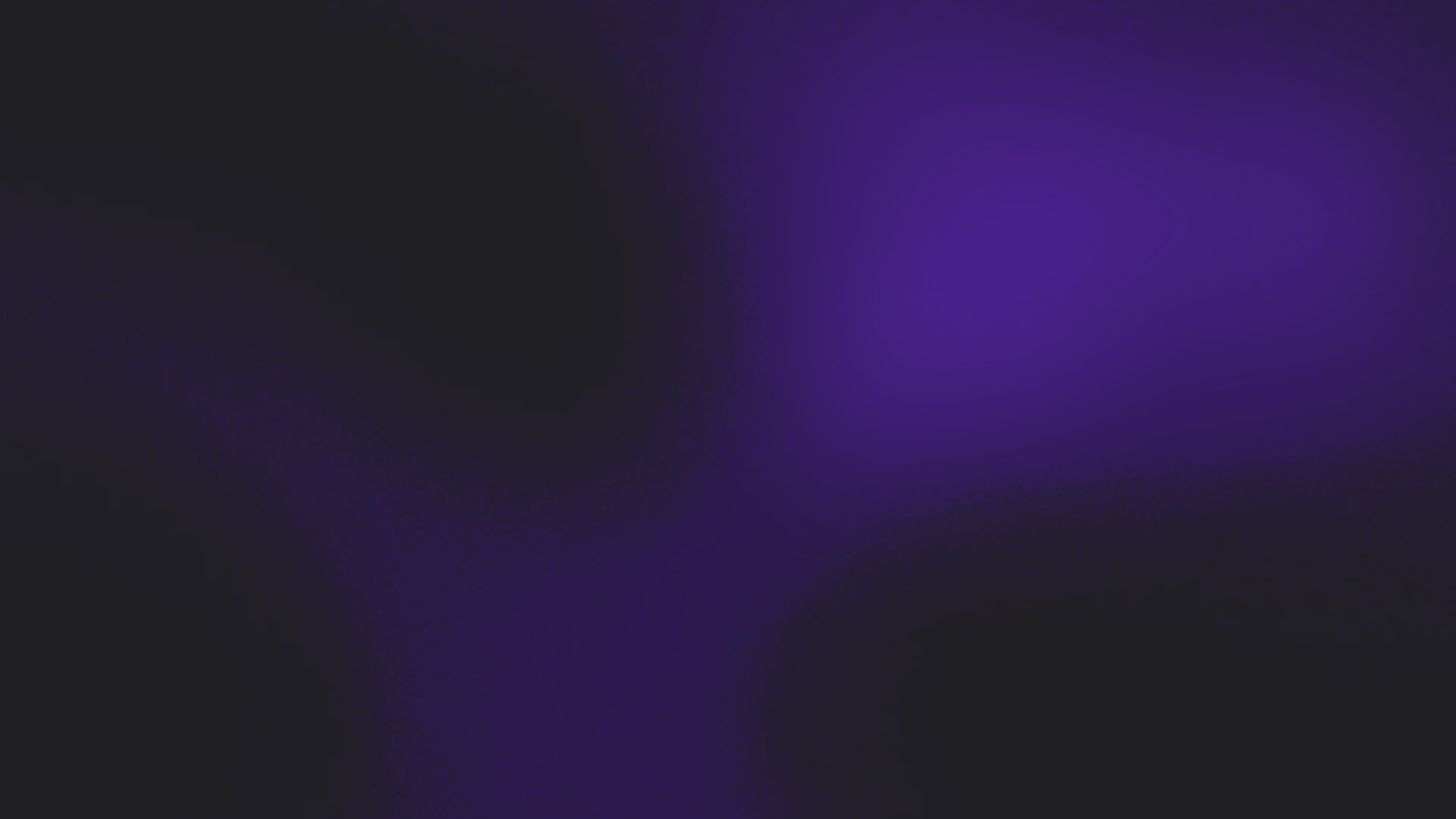